onClipEvent(enterFrame){
if(this.hitTest(_root._x=200,_root._y=200,true)){
this._y-= 5;
}
}
Okay, firstly, _root is simply a Movie Clip. It refers to the stage itself. You know how you can place symbols within symbols? Well, all of the flash game's symbols are being placed inside _root. So if you say _root._x = 200, you're going to move EVERYTHING over by 200, because you're changing the origin (anchor point) of the stage.
I think you may have meant "this._x", or just "_x"; in most cases both mean the same thing, and refer to the x-coordinate of which movie clip your function is written for. Or better yet, just use numbers:
this.hitTest(200,200,true);
That will check against the point at x=200, y=200.
Also, what specifically are you trying to collide? Sometimes, a bounding circle (a circle around the object that collides with other objects) can be MUCH better than a bounding box. It's also much easier to write yourself and much more efficient:
Say that every object has a radius r that you assign them. This is the radius of their bounding circle. To check whether two bodies overlap, all you need to do is check the distance between them against the sum of their radii; if the radii add up to more than the distance between them, then there's no way for them not to overlap. Sorry, this diagram should make it clearer:
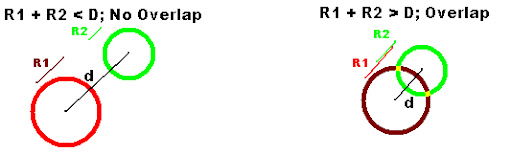
You could also set the radius up arbitrarily as half of the width of the MovieClip, so that you don't have to define variables. If you had one MovieClip named my_mc (I should say "if you had one reference to a MovieClip", but that's more technical Object-Oriented talk) and another named enemy_mc, the collision code within the my_mc object would look like:
onClipEvent(enterFrame) {
if(Math.sqrt((_x-_root.enemy_mc._x)^2 + (_y-_root.enemy_mc._y)^2) > (_width/2 + _root.enemy_mc._width/2)) {
//do this stuff if we overlap
}
}
This is pretty sloppy code; some easy optimizations would be to use ( )*( ) instead of ( )^2, because the exponent operator is slower than the multiplication one. Also, instead of ( )/2 + ( )/2 it could have been (( ) + ( ))/2. I just wrote it out so it would be easier to read.
So yeah, this uses a simple Pythagorean distance equation and checks it against the sum of their "radii". Circular bounding-boxes can provide some of the easiest and smoothest collisions you'd need, although they don't work in all occasions. If you want me to continue expounding on their use, let me know!